Transfers
Transfers occur between a source
and destination
payment method, which represents how and where money should move. The only other required field is an amount
.
|
|
Flow of funds
All transfers facilitated with Moov involve money moving in, out, through, or within the Moov platform. Since all funds flow through Moov, a single transfer can take advantage of multiple payment rails which allows you to tailor the cost, speed, and risk of each payment to your particular use case.
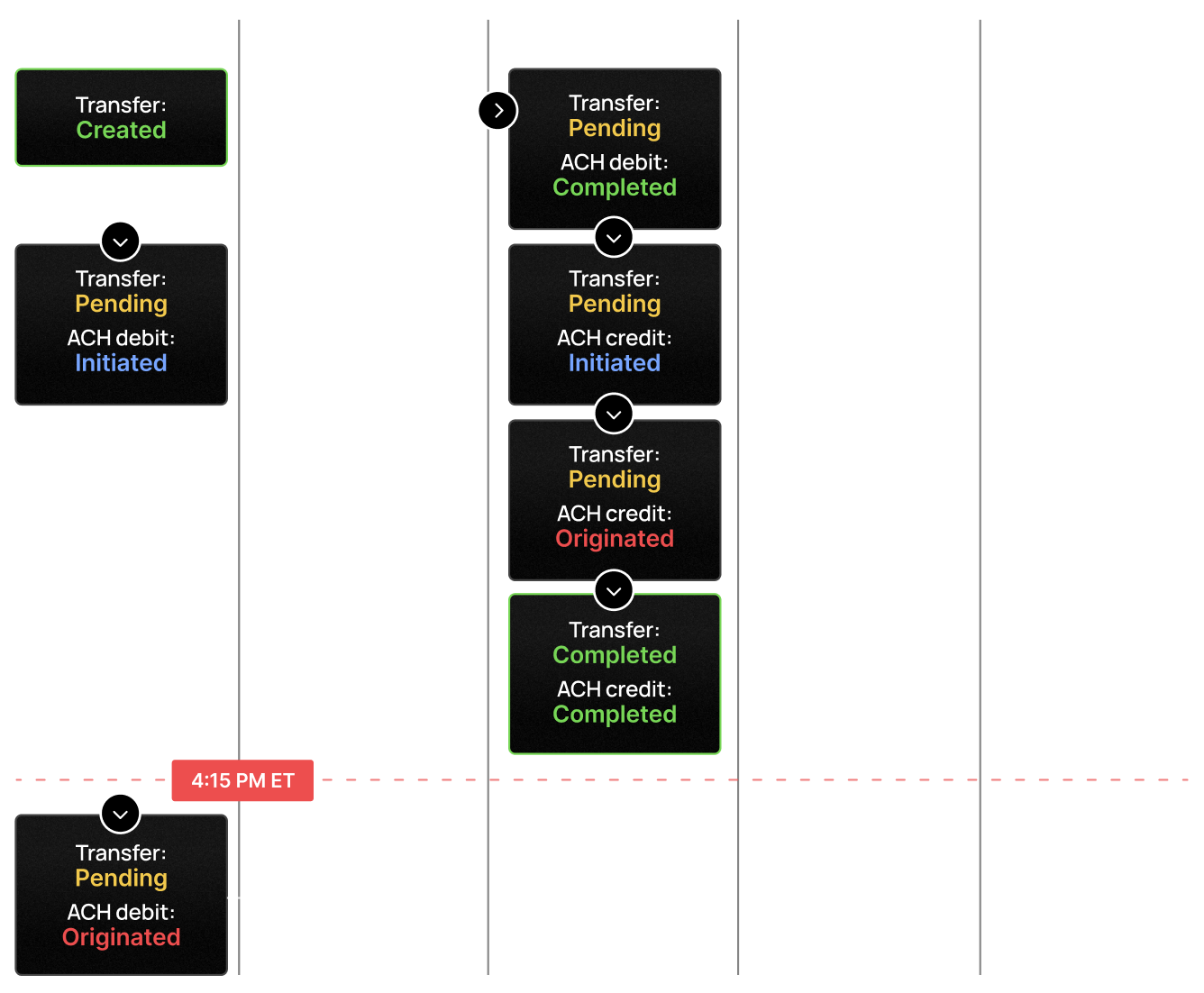
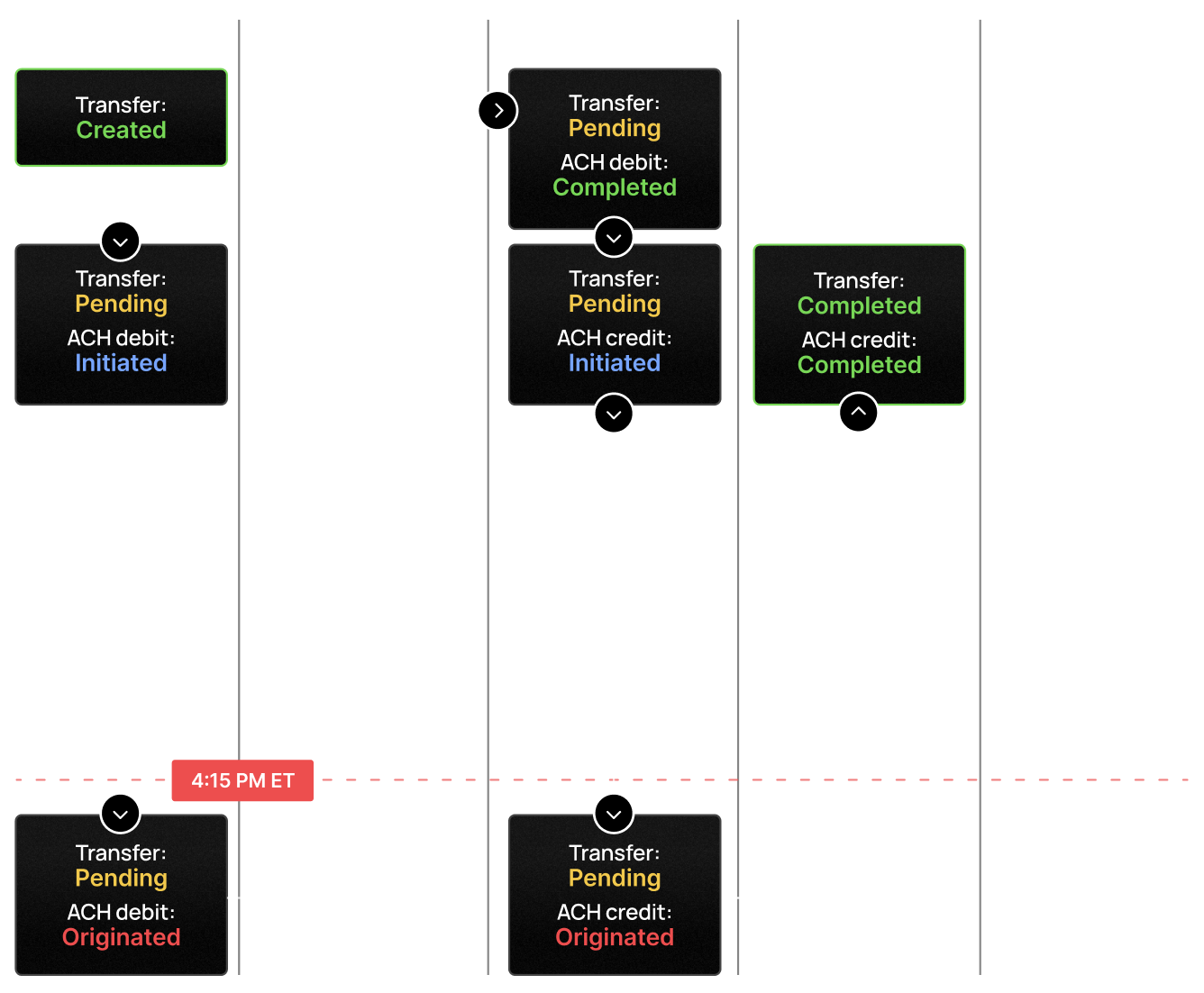
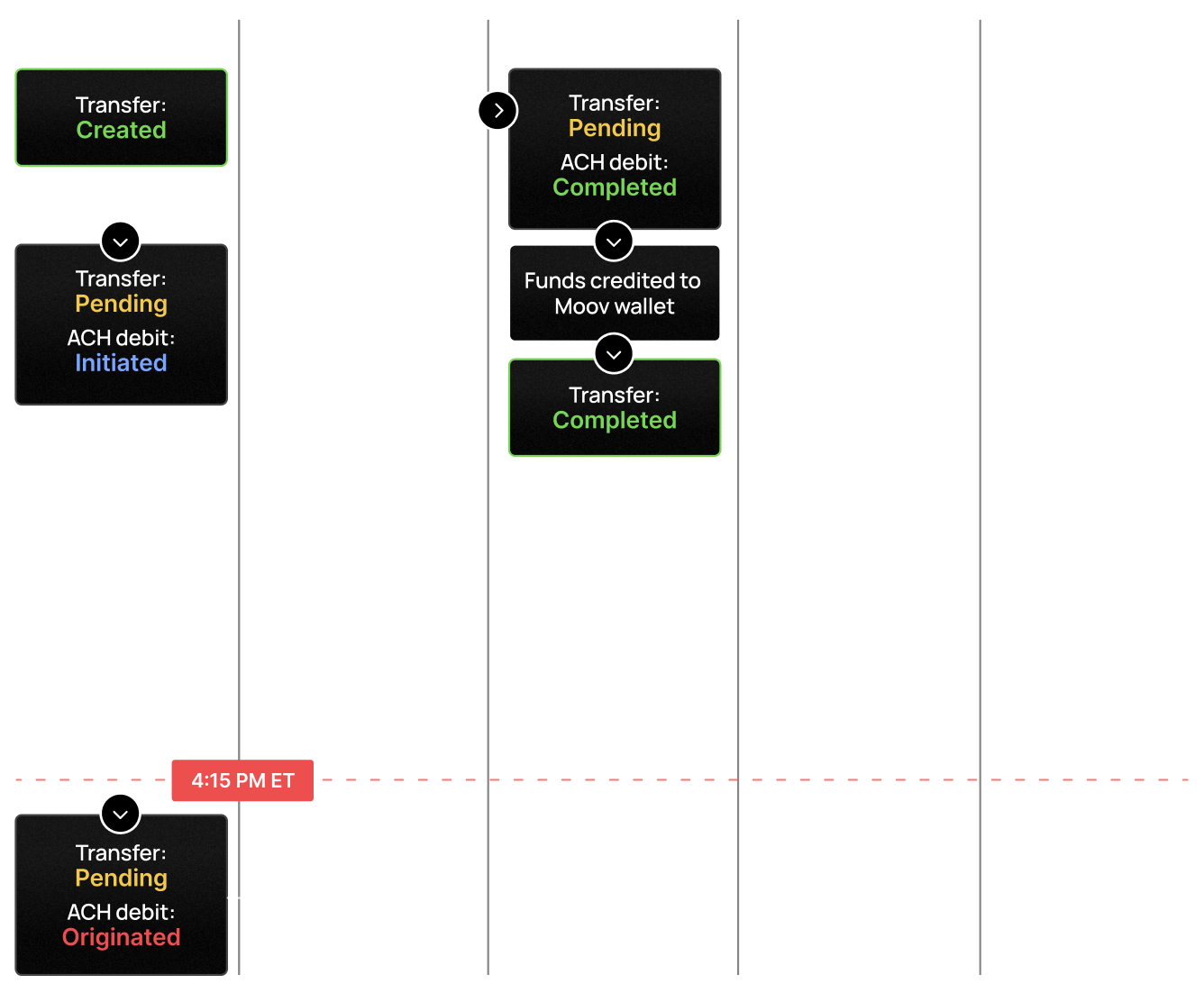
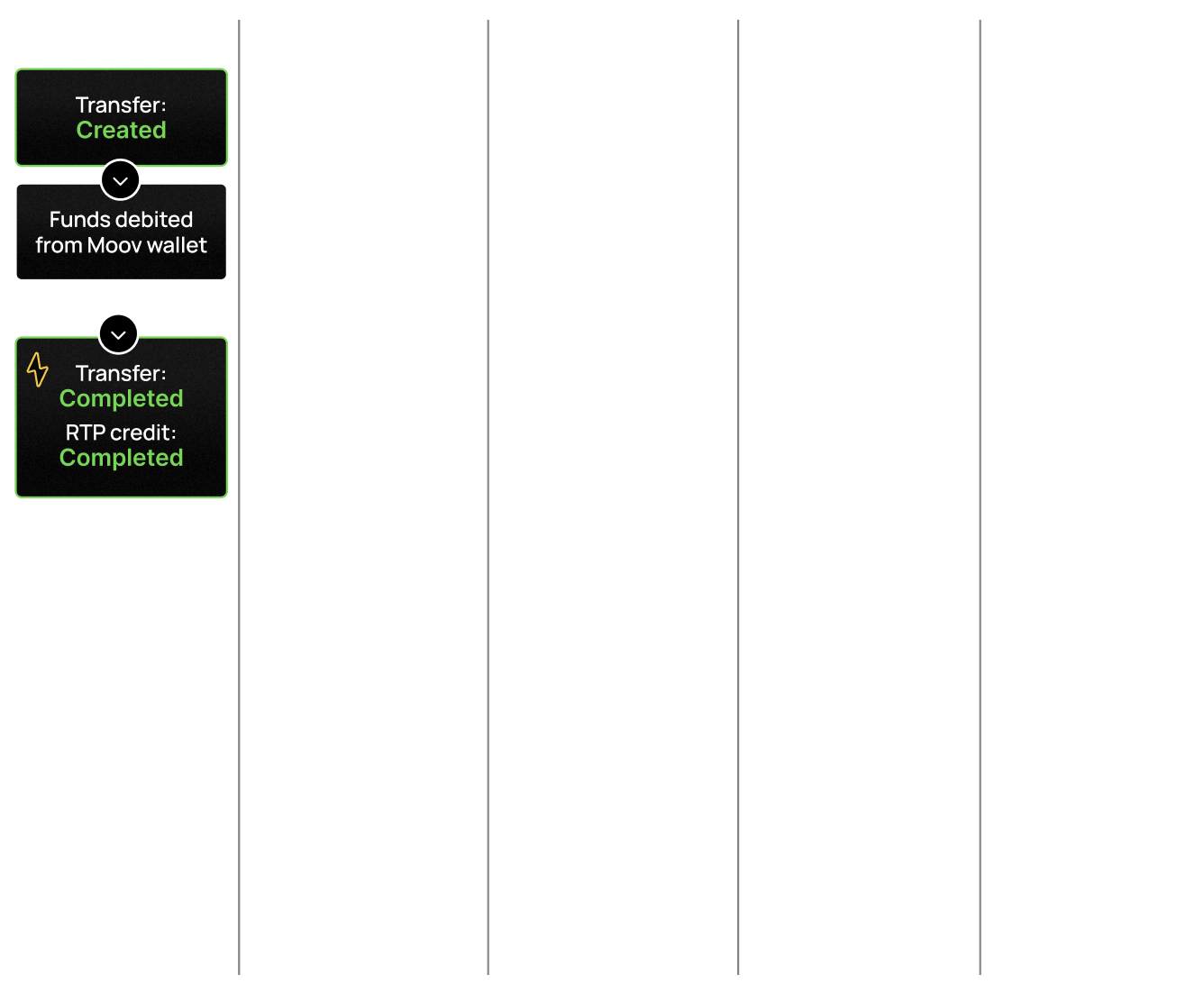
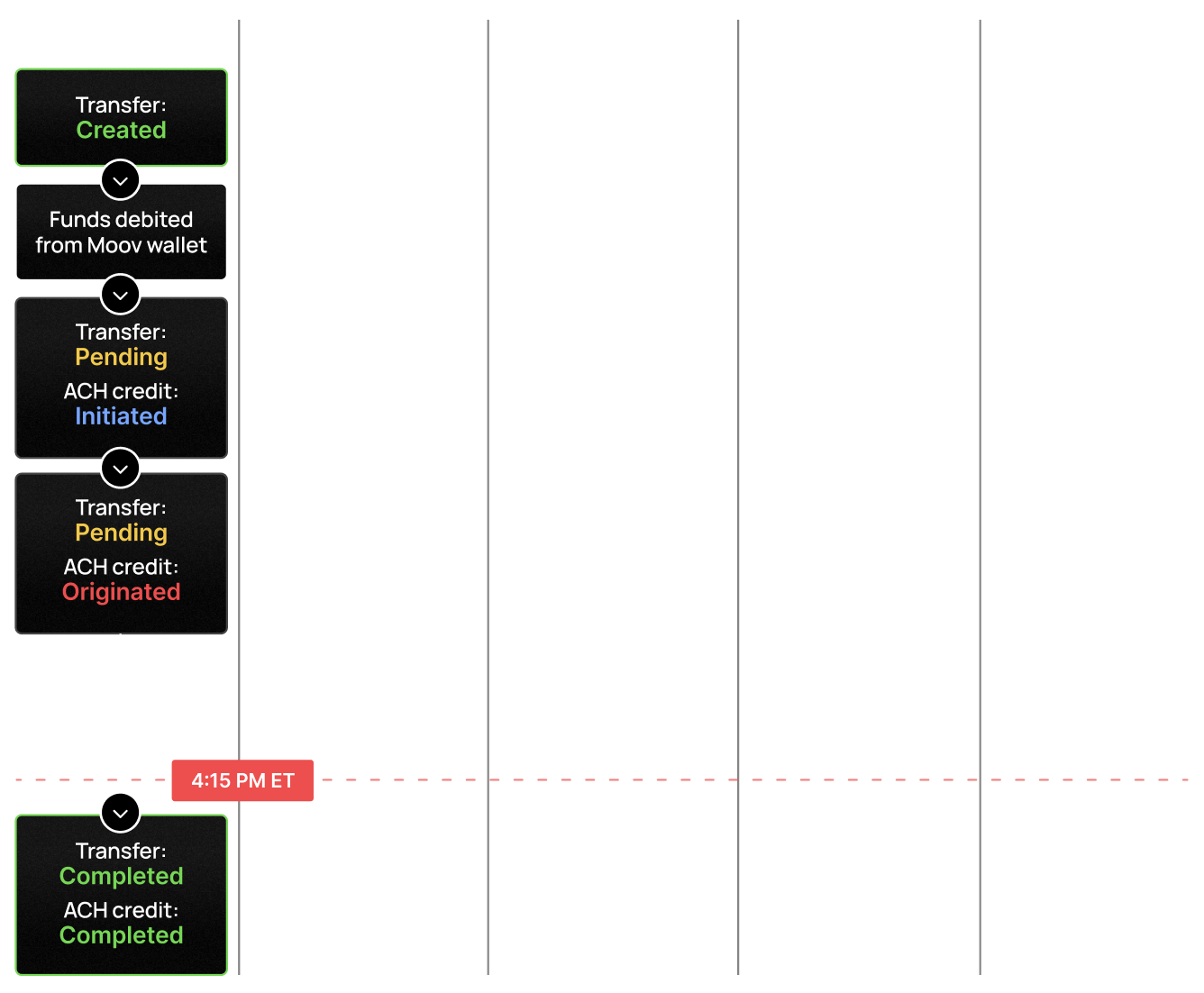
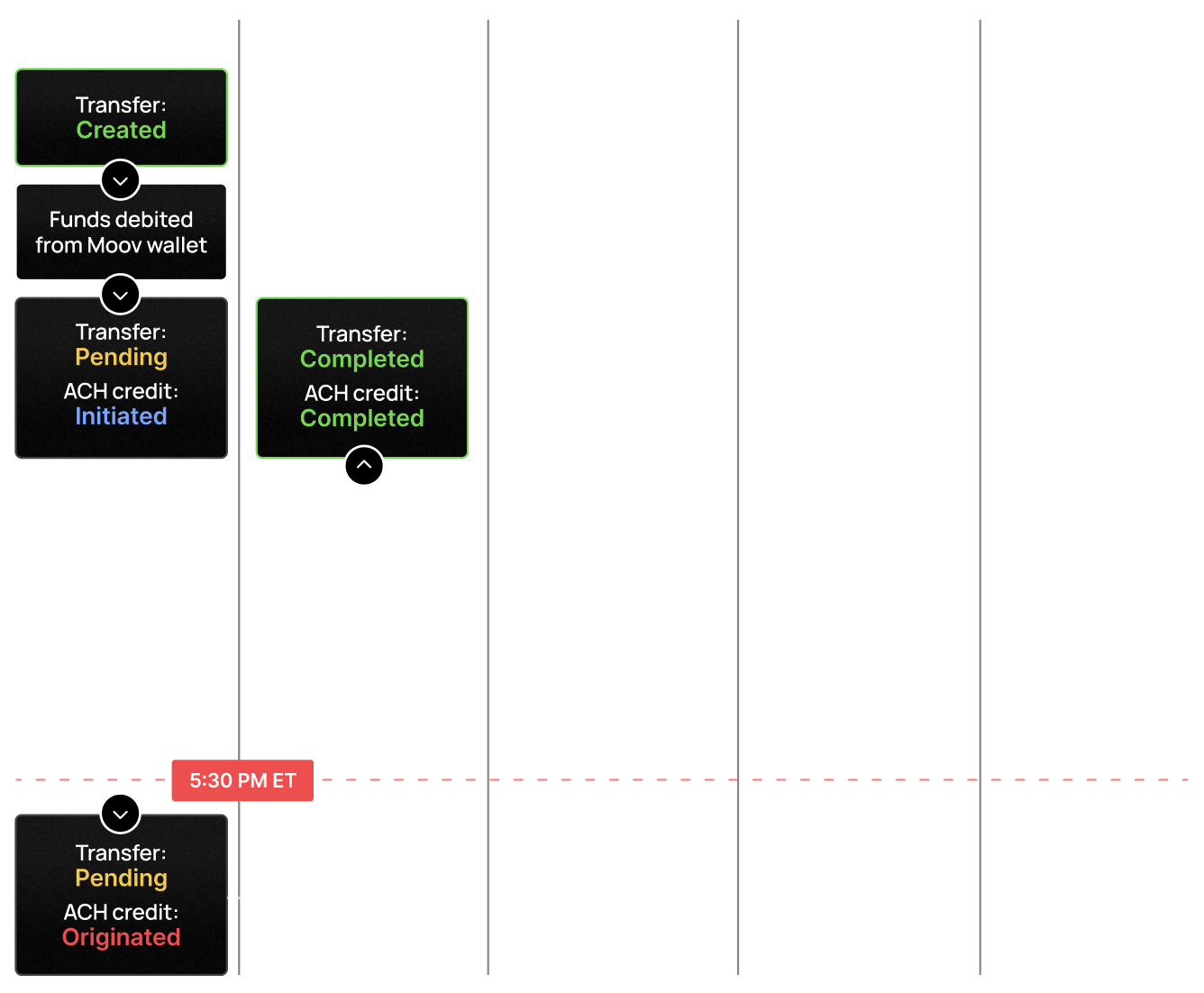
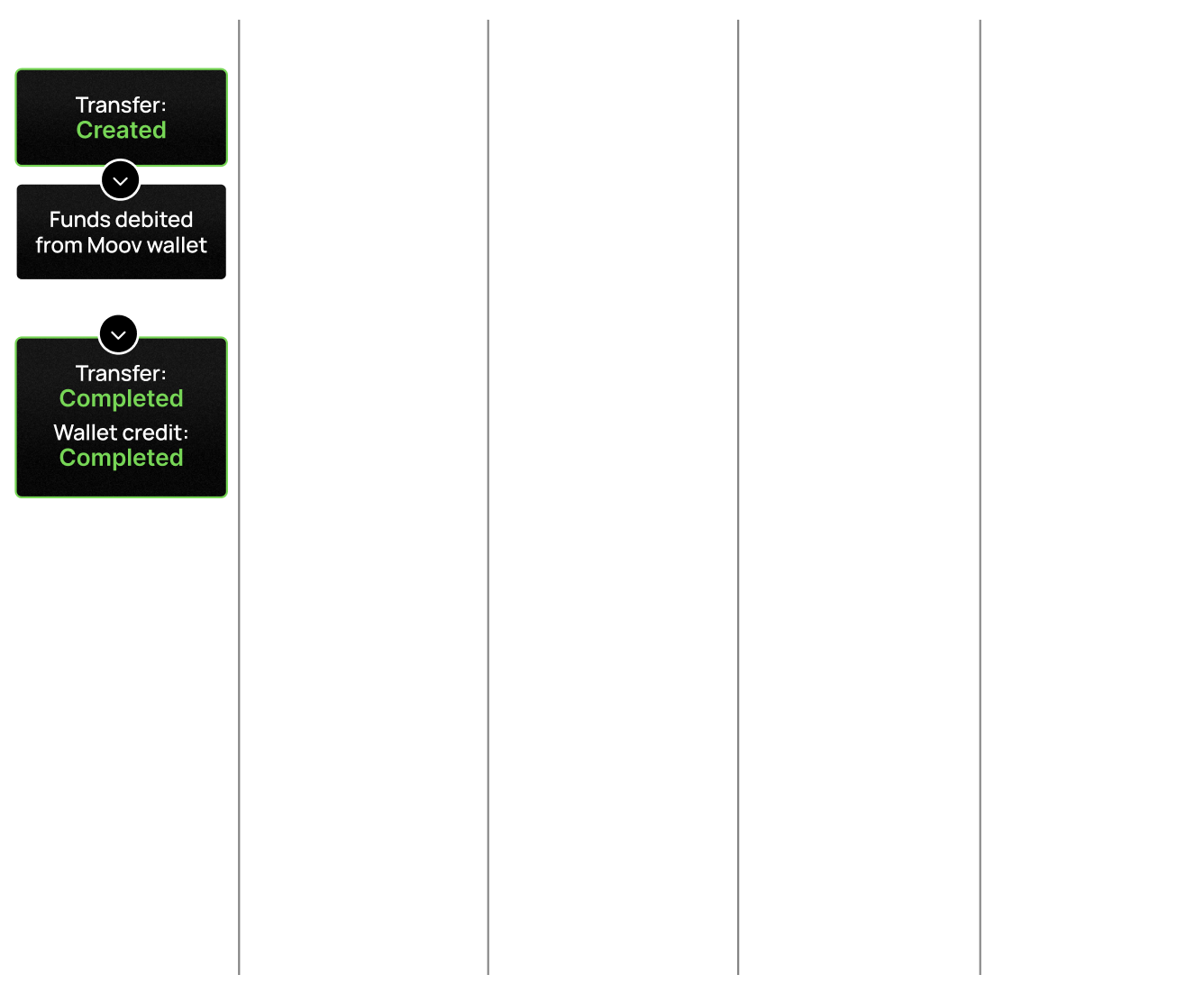
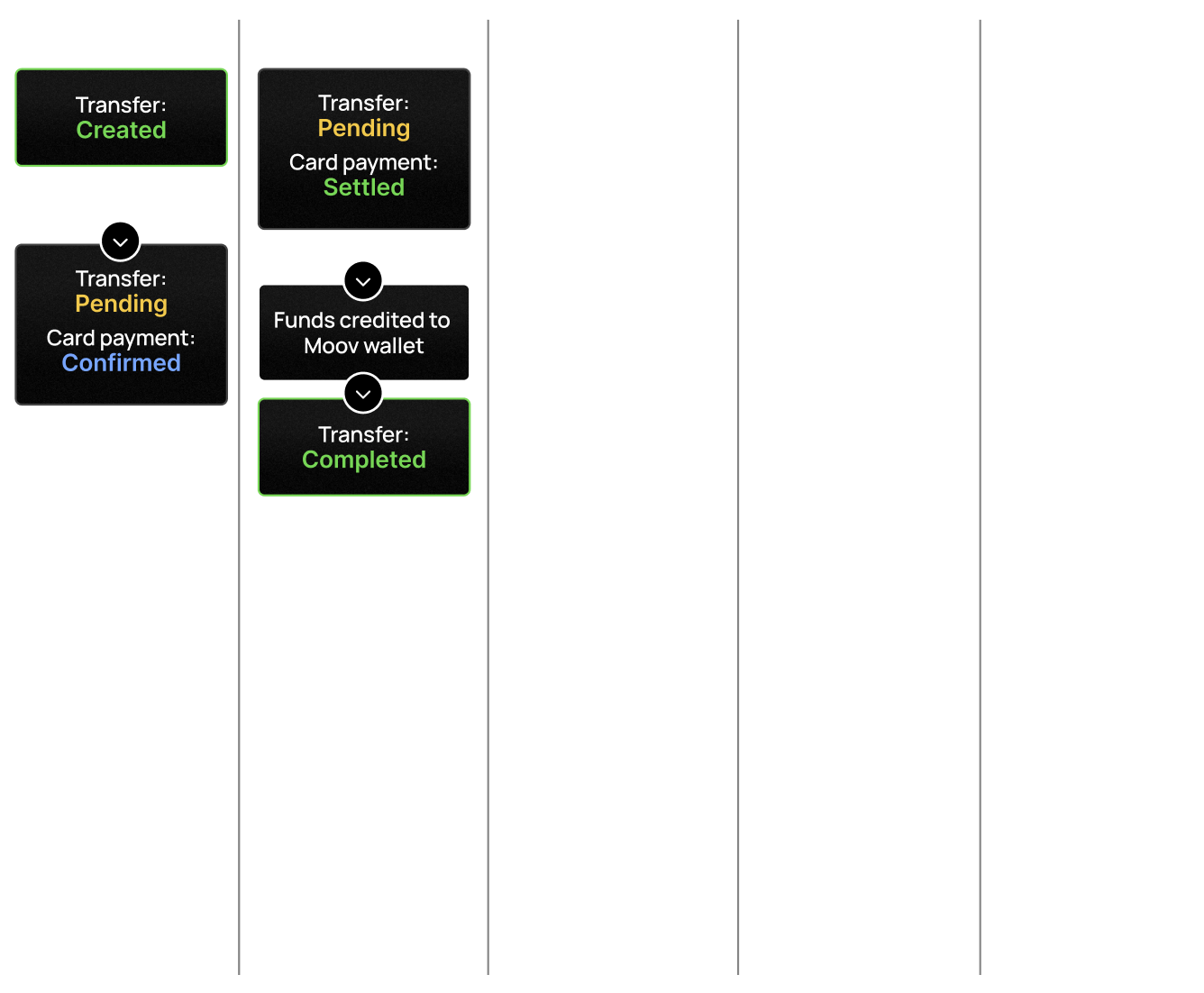
Transfer statuses
A transfer can have the following statuses:
Status | Description | |||
---|---|---|---|---|
queued |
A transfer that is part of a group will have the queued status until the preceding transfer has successfully completed | |||
pending |
The transfer is in progress, but not yet completed | |||
completed |
The transfer has completed | |||
canceled |
A transfer that is part of a group can fail and subsequent transfers will be marked as canceled | |||
failed |
The transfer did not succeed | |||
reversed |
The transfer completed, but funds were returned to the source |
Rail specific statuses are also available under the source and destination. You can view rail-specific statuses in our other guides:
Source
The source of a transfer represents the first stage of a transfer, when it is being funded. Transfers can be funded by external linked bank accounts, payment cards, or a Moov wallet balance. The following payment method types can be used as the source of a transfer:
ach-debit-fund
: fund payouts or add funds to a Moov wallet from a linked bank accountach-debit-collect
: pull funds from a linked bank account for bill payment, direct debit, or e-check type use-casesmoov-wallet
: fund a payout or withdraw funds from the Moov platformcard-payment
: initiate a payment from a linked credit or debit card
Destination
The destination represents the final stage of a transfer and the ultimate recipient of the funds. The following payment method types can be used as the destination of a transfer.
ach-credit-standard
: disburse funds to a linked bank accountach-credit-same-day
: disburse funds to a linked bank account using same-day processingrtp-credit
* : disburse funds to a linked bank account in near real timemoov-wallet
: add funds to a Moov wallet for multiple use cases
Transfer options utility
Moov offers the /transfer-options utility to provide you with an easy way to know which payment methods can be used in a transfer.
Some payment methods can only be used as the source or destination, and not all payment method combinations are valid options. The payment methods available for use also depend on the capabilities
of the source and destination accounts. Transfer-options
takes this business logic into account as well as network-specific restrictions such as maximum payment amounts to ensure a valid transfer request.
|
|
To use transfer-options, make a request to the /transfer-options
endpoint with a Moov accountID
or paymentMethodID
and an amount
.
- Supplying a single
accountID
in thesource{}
ordestination{}
: will return a list of available payment methods - Supplying an
accountID
for both thesource{}
anddestination{}
: will return two lists of payment methods filtered on the capabilities of the transfer participants - Supplying one
paymentMethodID
and oneaccountID
: will return a list of valid payment methods for theaccountID
provided
In practice, transfer-options can be used to present a menu of different options for how and where you want money to move.
FAQ
- Why did a transfer fail?
- Transfers can fail for a number of reasons related to the source or destination, or due to insufficient funds. Learn more through our guide on transfer failures.
- Can I re-start a transfer that never completed?
- Depending on the status of the transfer, you may be able to replay that transfer. Transfers in a “non-terminal” state (such as a child transfer stuck in
queued
) can be replayed by Moov, but transfers with thefailed
status are in a “terminal state”, meaning they cannot be replayed in our system. For reinitiating transfers that were in a terminal state, you will need to create a brand new transfer with the same details.